Register services and configure middleware
Startup.ConfigureServices method:
//Assume all resources are in the folder named "resources" in the website
services.AddLocalization(location => { location.ResourcesPath = "Resources"; });
services.AddSingleton<IStringLocalizerFactory, ResourceManagerStringLocalizerFactory>();
services.Configure<RequestLocalizationOptions>(
options =>
{
var supportedCultures = new List<CultureInfo>
{
new CultureInfo("en-CA"),
new CultureInfo("fr-CA")
};
//options.DefaultRequestCulture = new RequestCulture(culture: "en-CA");
options.DefaultRequestCulture = new RequestCulture(culture: "en-CA", uiCulture: "en-CA");
options.SupportedCultures = supportedCultures;
options.SupportedUICultures = supportedCultures;
//options.RequestCultureProviders.Insert(0, new QueryStringRequestCultureProvider());
//options.RequestCultureProviders = new List<IRequestCultureProvider>
// {
// new QueryStringRequestCultureProvider(),
// new CookieRequestCultureProvider()
// };
});
The current culture on a request is set in the localization middleware. The localization middleware is enabled in the Configure method of Startup.cs file.
app.UseRequestLocalization();
//var localizationOption = app.ApplicationServices.GetService<IOptions<RequestLocalizationOptions>>();
//app.UseRequestLocalization(localizationOption.Value);
then UseRequestLocalization
initializes a RequestLocalizationOptions
object. This should be placed at least before your UseMvc call.
Create resources
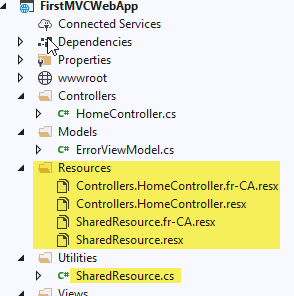
Inject the services
IStringLocalizer<T>
and/or IStringLocalizerFactory
implementation can be injected in the controller constructor through Dependency Injection:
private readonly ILogger<HomeController> _logger;
private readonly IStringLocalizer _controllerLocalizer;
private readonly IStringLocalizer _sharedLocalizer;
public HomeController(ILogger<HomeController> logger, IStringLocalizer<HomeController> localizer, IStringLocalizerFactory factory)
{
_logger = logger;
_controllerLocalizer = localizer;
var assemblyName = new AssemblyName(typeof(SharedResource).GetTypeInfo().Assembly.FullName);
_sharedLocalizer = factory.Create("SharedResource", assemblyName.Name);
}
The code above demonstrates each of the two factory create methods.
You can partition your localized strings by controller, area, or have just one container. In the sample app, a dummy class named SharedResource
is used for shared resources.
// Dummy class to group shared resources
public class SharedResource
{
}
To fully control the access to the resource, you can inject an IStringLocalizerFactory
into the controller or view.
Use the service
public IActionResult Index()
{
ViewData["HelloWorldFromControllerReource"] = _controllerLocalizer["HelloWorld"];
ViewData["HelloWorldFromSharedResource"] = _sharedLocalizer["HelloWorld"];
return View();
}
Change Culture
[HttpPost]
public IActionResult SetLanguage(string culture, string returnUrl)
{
Response.Cookies.Append(
CookieRequestCultureProvider.DefaultCookieName,
CookieRequestCultureProvider.MakeCookieValue(new RequestCulture(culture)),
new CookieOptions { Expires = DateTimeOffset.UtcNow.AddYears(1) }
);
return LocalRedirect(returnUrl);
}
Get the current language
var currentLanguage = HttpContext.Features.Get<IRequestCultureFeature>().RequestCulture.Culture.Name;